Last years approach in developing and delivering software to the customer is so popular Docker. Docker allows us to lock our software in some box in some environment and that environment is static and called - container. It means that there is already configured all what is required for our software. This is very good situation, because you don't have make huge installation instruction for your client or customers. In this article I will show you how to build Docker container and start there Symfony 5.0 application.
Lets get it done, but what we should start from?
We will start from installation Docker on your workstation to be able work with containers.
My example is based on Ubuntu 18.04 workstation. So first lets install docker running command in terminal: sudo apt-get install docker
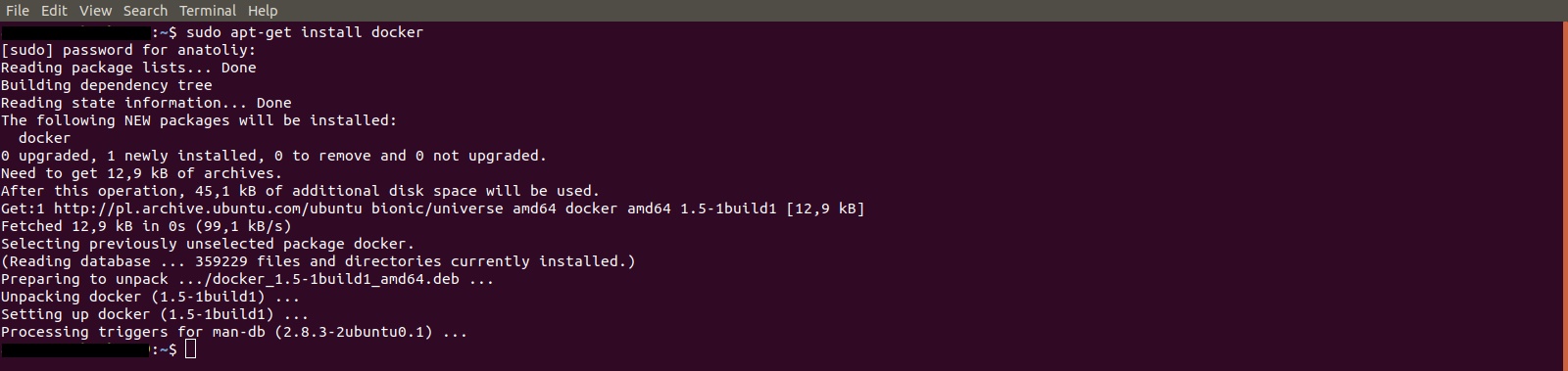
The next step is to install docker-compose. As for me installation worked without following official instructions from here https://docs.docker.com/compose/install/ It was enough to type sudo apt-get install docker-compose sudo apt install python3-pip sudo pip3 install docker-compose on Ubuntu 18.04 terminal and it was installed.
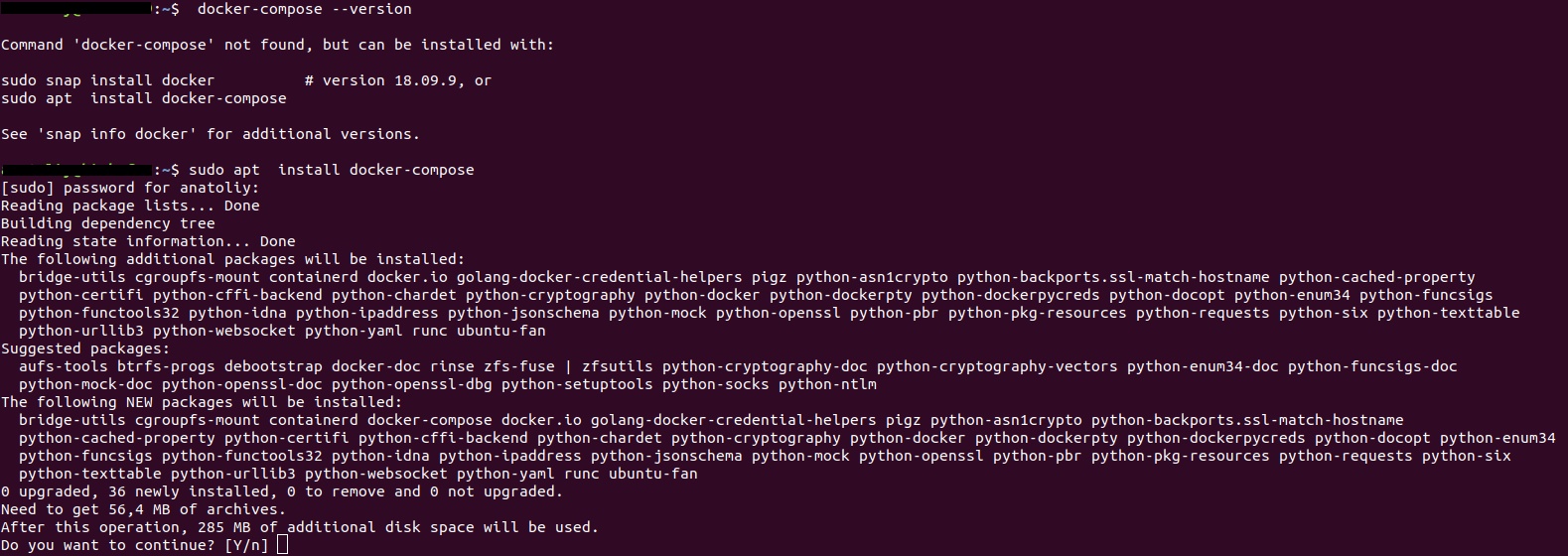
To verify if docker-composer installed and proper version - type: docker-compose --version
I've got this: docker-compose version 1.17.1, build unknown
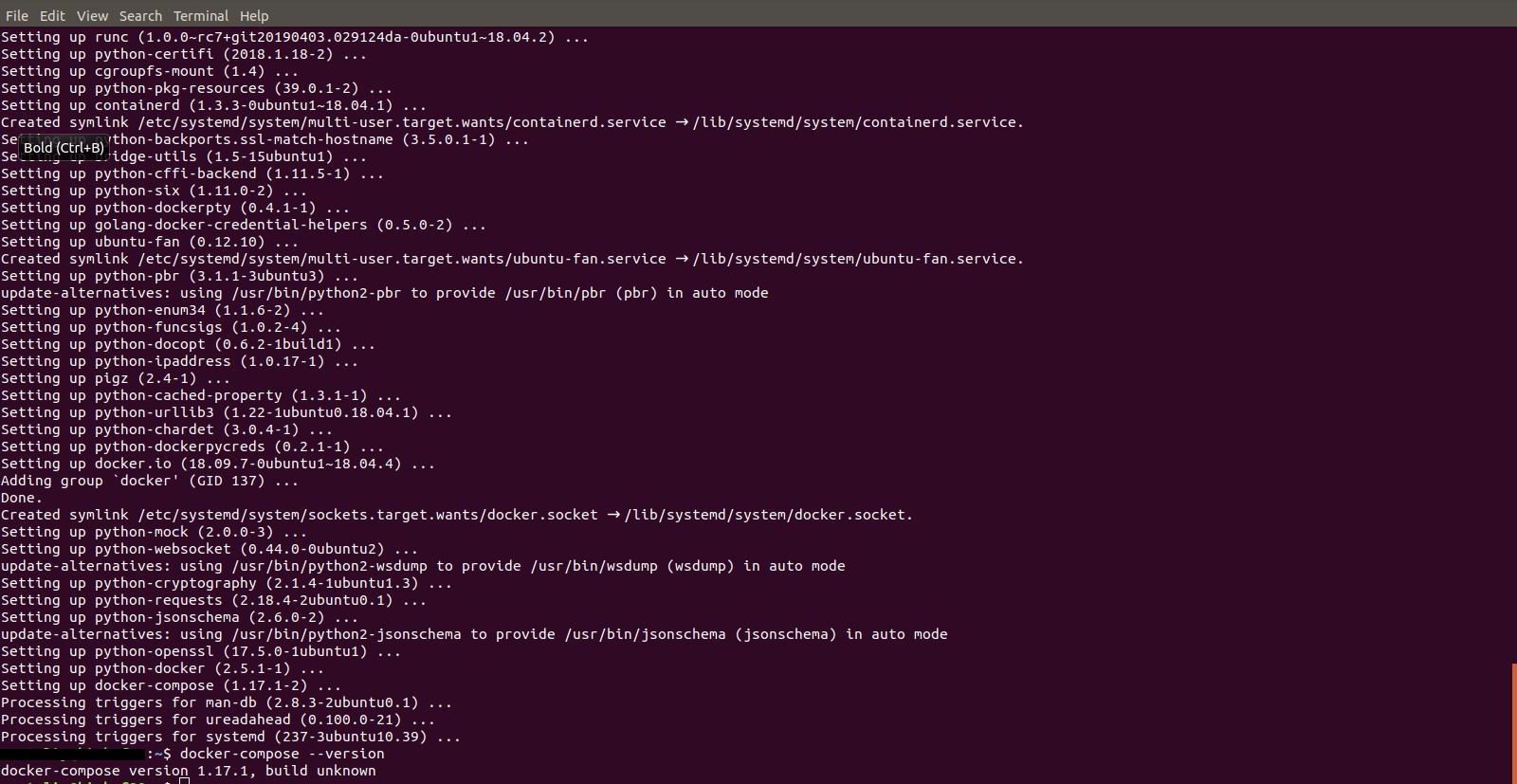
Environment is ready, so whats next!?
I will omit questions about configuring new project in IDE and etc. Now it is time to build your Dockerfile. What is Dockerfile? So it is special file that allow you using docker instructions build proper environment and run the project. My Dockerfile instructions looks like this:
FROM ubuntu:18.04
ENV TZ 'Europe/Warsaw'
##Timezone
RUN echo $TZ > /etc/timezone && \
apt-get update && apt-get install -y tzdata && \
rm /etc/localtime && \
ln -snf /usr/share/zoneinfo/$TZ /etc/localtime && \
dpkg-reconfigure -f noninteractive tzdata && \
apt-get clean
#Update and install some PHP/DB stuff
RUN apt-get update
RUN apt-get install -y software-properties-common sudo \
&& add-apt-repository ppa:ondrej/php \
&& apt-get update \
&& apt-get install -y \
php7.4 \
php7.4-dom \
php7.4-curl \
php7.4-fpm \
php7.4-gmp \
php7.4-common \
php7.4-mysql \
php7.4-cli \
php-pear \
php7.4-dev \
php7.4-zip \
php7.4-sqlite \
mysql-client \
vim \
nano \
mc \
nginx-full \
git \
curl \
acl \
composer \
htop \
&& apt-get remove --purge -y $BUILD_PACKAGES \
&& rm -rf /var/lib/apt/lists/* \
RUN a2enmod php7.4 \
#Some trick to install curl
RUN apt remove libcurl4 \
&& apt install -y libcurl4 curl
RUN apt-get update \
&& apt-get install -y autoconf g++ make openssl libssl-dev libcurl4-openssl-dev libcurl4-openssl-dev pkg-config libsasl2-dev \
&& apt-get remove --purge -y $BUILD_PACKAGES \
&& rm -rf /var/lib/apt/lists/*
#Install Forego to keep docker running
RUN curl -O https://bin.equinox.io/c/ekMN3bCZFUn/forego-stable-linux-amd64.tgz \
&& tar -xzf forego-stable-linux-amd64.tgz -C /usr/bin \
&& rm forego-stable-linux-amd64.tgz \
&& mkdir -p /run/php
# Configure php.ini
RUN echo "cgi.fix_pathinfo = 0" >> /etc/php/7.4/fpm/php.ini
RUN echo "memory_limit = 1024M" >> /etc/php/7.4/fpm/php.ini
RUN echo 'date.timezone = "Europe/Warsaw"' >> /etc/php/7.4/fpm/php.ini
#RUN echo 'variables_order = "EGPCS"' >> /etc/php/7.4/fpm/php.ini
# Configure www.conf
RUN echo "catch_workers_output = yes" >> /etc/php/7.4/fpm/pool.d/www.conf
RUN sed -e 's/;clear_env = no/clear_env = no/' -i /etc/php/7.4/fpm/pool.d/www.conf
RUN sed -e 's/max_execution_time = 30/max_execution_time = 120/' -i /etc/php/7.4/fpm/php.ini
RUN sed -e 's/pm.max_children = 5/pm.max_children = 10/' -i /etc/php/7.4/fpm/pool.d/www.conf
# Copy configuration
COPY docker/nginx.conf /etc/nginx/sites-available/default
COPY docker/nginx_server.conf /etc/nginx/nginx.conf
#COPY docker/Procfile /Procfile
# Copy docker entry point script
COPY docker/start_docker.sh /docker/start_docker.sh
RUN chmod -R 0755 /docker/start_docker.sh
# Copy application source
COPY . /var/www/html/
#move permissions to www-data
RUN chown www-data:www-data /var/www/html -R
RUN ls -la /var/www/html
EXPOSE 80
ENTRYPOINT /docker/start_docker.sh
Lets see another configurations file and real deploy on next part of article.
Want to take a look on code on Gitlab?
No problem - you can go to my repo on Gitlab and check the code:
https://gitlab.com/birkof89/symfony5-on-nginx-php-fpm-under-docker