What are benefits from reading this article?
I will share with you my approach to solve elevator system design. For sure it will be very useful for you to study new design or enrich your knowledge in case you already had an experience to solve this type of design.
Advantage of my design is that it will work for multiple elevators. So best choice selection solution will be implemented.
Problem to solve: an multiple elevators system design based on object-oriented principles.
Elevator state
Let's start our thinking from elevator states. We can define three states of elevator: moving up, moving down and idle. In fact when elevator stops - it is a part of moving process up or down. The best way of representing those states is enum. This how it looks like:
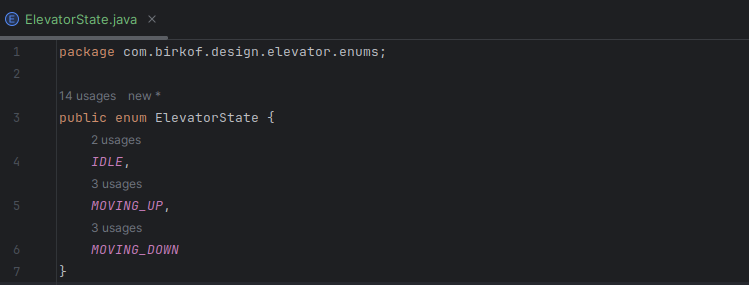
Elevator class
In the Elevator class I will prepare most of main functionalities and parameters of real elevator. Here we need to have state parameter to know elevators current state - is it moving down or up or, maybe, it is idling. Next parameter is currentFloor. This parameter will help us to calculate time needed to reach desired floor from current elevator state and choose fastest one.
Of course, I need to have stopFloorList parameter and I have it as LinkedList data type. You may find in other solutions that PriorityQueue is used, but, in my opinion, it is an mistake. In fact we need extra logic when adding new elevator stop to the stop list and it depends from elevator state and current floor.
A bit complex logic of assigning new stop will be realized in addStop() method. Pay attention to that method and make sure you understood the logic there.
As I mentioned before - I need to choose fastest elevator to come, so I add getReachingFloorTime() method. This method will return us time, needed by elevator, to finish it's current tasks and come to desired floor for pickup. Of course, if elevator is moving thru desired floor - it can stop and pickup on desired floor. Pay attention to that method and make sure you're fully okay with that logic.
Elevator class code listing:
Elevator class implements Serializable, because I'm going to implement mechanism of storing and restoring each elevator state. It is must-have functionality, I'd say even critical functionality for cases of power outage. Nobody want to stay immobilized in the elevator in case of even short power outage.
I will realize this functionality in the ElevatorController class.
ElevatorController class
First of all I'm going to prepare system initialization with n elevators at start. This is realized in ElevatorController constructor.
Method pickupElevator() is for pressing elevator button outside the elevator. It calculates time of arrival for each elevator and assigns the call to fastest elevator. On other side addStopInternally() will add new stop for current elevator.
For both methods logic will work in the way when it will add new stop to the middle of the list if stop floor is on the elevators way or it will add new stop to the end of elevators stop list.
Lets see ElevatorController class code listing:
Methods saveElevatorsState() and restoreElevatorsState() allow us to store Elevator objects to the hard drive or other storage and, if needed, restore Elevator objects with latest state. It allows to keep elevators working even after short power outage or other failure.
Where you can find source code from this article?
You can find source code from this article on my Gitlab repo, just follow link below:
https://gitlab.com/birkof89/designs-java
What about tests?
Correct, test are integral part of software development process. However, I will be doing tests along with discussing the design source code on the video I prepared on my YouTube. You can find the link to video at the bottom of this article.